Overview
ClassRepO is a desktop address book application written in Java that targets secondary schools for usage by their students, tutors and administrative staff. This portfolio is meant to document the contributions that I made to the project.
Roles
In this project, I serve mainly as a Code Quality checker. Aside from the code quality, I also ensure that the language used in the documentation is up to standard.
Summary of contributions
-
Code Contributions: here
-
Major enhancement: added a new field Attendance which comes with a few commands
-
What it does: Enables the ClassRepO to keep track of the students' attendance.
-
Justification: This feature improves the product significantly as a school already needs to take the students' attendance. A digitalized storage and management of the attendance will make data analysis faster and also reduces the usage of paper (eco-friendly).
-
Highlights: This feature uses different forms of software tricks (storage type) to make searching and editing of data more efficient.
-
Credits: Victor Hernandez for the base valid date checker method
-
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Attendance Commands
Below are the commands that deal with attendance data:
Updates the attendance of a person: attendance

Updates the attendance of the target person.
Format: attendance INDEX d/DATE att/ATTENDANCE
Example(s):
-
list
attendance 1 d/29-09-2018 att/1
Marks the attendance of the first person in the list as present for the date 29th September 2018. -
list
attendance 1 d/0 att/0
Marks the attendance of the first person on the list as absent for today’s date.
Replaces the attendance of a person: replaceAtten

Replaces the current attendance of the target person.
Format: replaceAtten INDEX d/DATE att/ATTENDANCE
Example(s):
-
list
replaceAtten 1 d/29-09-2018 att/1
Replaces the attendance of the first person in the list as present for the date 29th September 2018.
View the attendance of a person: viewAttenPerson

View the attendance of the target person.
Format: viewAttenPerson INDEX
Example(s):
-
list
viewAttenPerson 1
Views the attendance of the first person on the list.
View the attendance of a date: viewAttenDate

View the attendance of the given date.
Format: viewAttenDate DATE
Example(s):
-
viewAttenDate d/28-10-2018
Shows a list of people who were present on a particular date. -
viewAttenDate d/0
Shows a list of people who were present today.
Replaces the attendance of a person: replaceAtten

Replaces the current attendance of the target person.
Format: replaceAtten INDEX d/DATE att/ATTENDANCE
Example(s):
-
list
replaceAtten 1 d/29-09-2018 att/1
Replaces the attendance of the first person in the list as present for the date 29th September 2018.
Update multiple people attendance: multiAtten

Updates the attendance of multiple people based on the list of index.
Format: multiAtten i/MULTIPLE_INDEX d/DATE att/ATTENDANCE
Example(s):
-
list
multiAtten i/1 2 4 5 d/29-09-2018 att/1
Marks the attendance of the people with index 1, 2, 4 and 5 in the list as present for the date 29th September 2018. -
list
attendance i/0 d/0 att/0
Marks the attendance of everybody on the list as absent for today’s date.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Attendance Feature
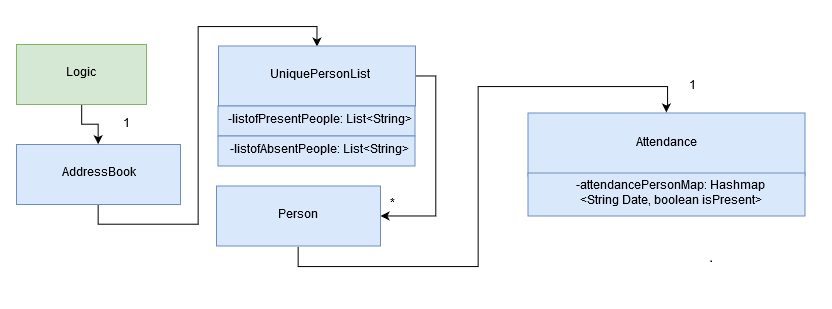
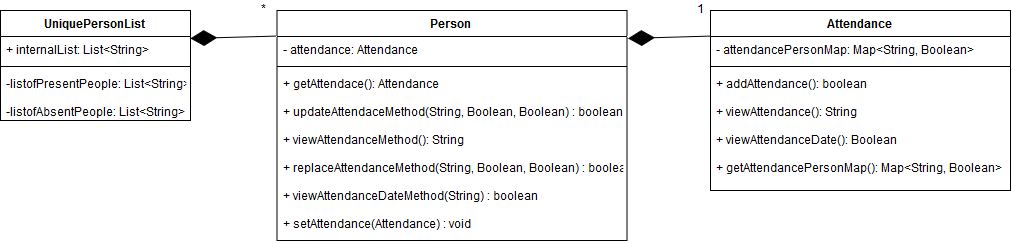
The attendance field is an additional field to every Person
object:
-
Each
Person
will have anAttendance
object -
Each
Attendance
object will contain a Hashmap, which will store the attendance of each person by keying each Stringdate
to a booleanisPresent
. -
UniquePersonList
will contain 2 lists, containing a list of people who are present or absent for each particular date. -
Users can thus check the attendance of a particular person, or for a particular date.
Current Implementation
The AddressBook contains the attendance of each person for each date that his/her attendance is taken. The current set of commands include:
-
Updating a person’s attendance
-
Replacing a person’s attendance
-
Viewing of a person’s attendance
-
Viewing of present people on a particular date
An example of how feature 1 - Updating a person’s attendance
works:
-
The user (teacher/ admin) will be able to use the 'attendance' command to update the attendance of a particular person (student).
-
The specific person is extracted from the AddressBook.
-
A check is performed to check if the person already has his/her attendance taken.
-
If the attendance has already been taken, the user will be prompted to use another command
replaceAtten
to replace the attendance. -
If the attendance has yet to be taken, the attendance of the specified person for the specified date will be taken as either 'present' or 'absent'.
Design Considerations
Aspect: How to store attendance for each person
-
Alternative 1 (Current choice): Each person has a hashmap that stores the date to the attendance.
-
Pros: Hashmaps allows more efficient checking of duplicate attendance (get() method has a complexity of O(1).
-
Cons: Values and Keys in hashmap are not sorted.
-
-
Alternative 2: Each person has a list of strings containing the date and attendance.
-
Pros: A list of strings will allow easier storage and viewing of the data
-
Cons: List or ArrayList will require O(n) time to check for duplicates
-
Cons: A single string will not allow handling of data separately
-
-
Alternative 3: Each person has a list of pairs containing date and attendance.
-
Pros: Pairing of date to attendance ensures that the checking of attendance requires at most O(n) time, but minimally O(1)
-
Cons: Usage of pairs in java will require an extra class or an external library, which is unnecessary
-
Aspect: How to check if attendance has duplicate
-
Alternative 1 (Current choice): Use a boolean to check if there is a duplicate date in the hashmap.
-
Pros: Booleans only have 2 value, and thus there is no need to define a string with a proper variable name
-
Cons: Booleans are fixed as true and false, thus needed additional code to translate the result into a string so that the user can understand the results easily.
-
-
Alternative 2: Use a separate class, 'hasDuplicate' to check for duplicate date
-
Pros: An additional class means that the code will be more encapsulated, fulfilling the purpose of OOP.
-
Cons: Additional code will be required to create the additional class
-
Aspect: How to differentiate between updating and replacing a person’s attendance
-
Alternative 1 (Current choice): Using an additional parameter (Boolean overWrite) that is fixed in the command.
-
Pros: No confusion for the user since the parameter to overwrite is fixed.
-
Pros: THere is no need to have 2 separate methods in the Attendance object as both updating and replacing of attendance has very similar functions.
-
Cons: There is a need to check for an additional parameter in the same method, which may lengthen the code and cause minor 'arrowhead' coding.
-
-
Alternative 2: Treat the 2 commands as 2 completely separate commands and execute them individually.
-
Pros: The 2 commands will each be short, simple and easy to understand.
-
Cons: There will be 2 methods with extremely similar functions, which is redundant.
-
-
Alternative 3: Have the user input an additional parameter (Boolean overWrite) if they want to overwrite the current data
-
Pros: Similar to alternative 1, having the user input an additional parameter and only having 1 method in the Attendance object can reduce the need to have unnecessary duplicates in the code.
-
Cons: An additional parameter will require a change in the parser.
-
Aspect: How to store data in XML, addressbook.txt
-
Alternative 1 (Current choice): Use 2 list to store a list of dates and a list of absent/present
-
Pros: Simple implementation, and utilizes the fact that each date can only have either present/absent. Iterating through the map to generate the 2 lists will thus ensure that the data is in order
-
Cons: In the XML format, data will be stored as 2 separate lists, which may not be as user-friendly to edit directly.
-
-
Alternative 2: Use a list of pairs, Pair <String Date, Boolean isPresent>
-
Pros: Only requires 1 single list to store the data of the entire map.
-
Cons: Such implementation will require a Pair object, which will require additional methods and classes, thus requiring longer code.
-
-
Alternative 3: Convert Hashmap to XML directly
-
Pros: Direct transfer of data will thus require less memory space to store the same data.
-
Cons: A Hashmap to XML converter will require either a very long code (unnecessary for only 1 map), or requires the import of an external library.
-
Coming in v2.0
Aspect: : How to implement multiAtten
Command
-
Alternative 1 (Most likely choice):
multiAtten
command calls theattendance
command multiple times-
Pros: Reduce the need for repeated code since the multiAtten command is very similar to attendance command.
-
Pros: Simpler to implement when the input is 0 as there is no need to catch index 0 since index 0 will not exist in the UniquePersonList.
-
Cons: Increase in coupling as a change in
attendance
command will causemultiAtten
command to change.
-
-
Alternative 2 (Unlikely choice): Reimplement the command as a brand new command
-
Pros: Cleaner codes
-
Pros: A brand new implementation would allow for future upgrades
-
Cons: Lengthier and repeated code
-